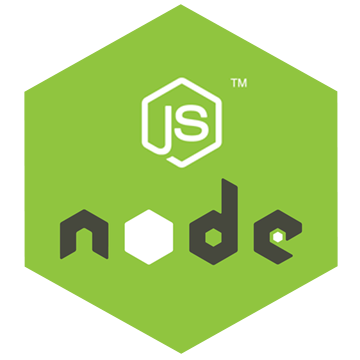
Create a react application
c:\ npx create-react-app my-app
If the creation of react app is generating error like ‘Unexpected end of JSON input while parsing near …’
c:\ npm cache clean –force
Correct way of updating the state variable
this.setState((state, props) => ({ counter: state.counter + props.increment }));
this.setState(function(state, props) { return { counter: state.counter + props.increment }; });
When we would need to return multiple <td>
elements in order for the rendered HTML to be valid. If a parent div was used inside the render()
, then the resulting HTML will be invalid.
<table>
<tr>
<div>
<td>Hello</td>
<td>World</td>
</div>
</tr>
</table>
To avoid this, use Fragment
function ListItem({ item }) {
return (
<Fragment>
<td>{item.term}</td>
<td>{item.description}</td>
</Fragment>
);
}
Run node server.js that is in a different folder
c:\ node d:\node\server.js
Run npm start that is in a different folder
c:\ npm start --prefix d:\node\
Run npm on port 80
“scripts”: {
“start”: “set PORT=80 && react-scripts start”,
“build”: “react-scripts build”,
“test”: “react-scripts test”,
“eject”: “react-scripts eject”
},